Let's try adding a popup to the button. Replace manifest.json with this:
{
"description": "Demonstrating toolbar buttons",
"manifest_version": 2,
"name": "button-demo",
"version": "1.0",
"browser_action": {
"browser_style": true,
"default_popup": "popup/choose_page.html",
"default_icon": {
"16": "icons/page-16.png",
"32": "icons/page-32.png"
}
}
}
We've made three changes from the original:
- We no longer reference "background.js", because now we're going to handle the extension's logic in the popup's script (you are allowed background.js as well as a popup, it's just that we don't need it in this case).
- We've added
"browser_style": true
, which will help the styling of our popup look more like part of the browser.
- Finally, we've added
"default_popup": "popup/choose_page.html"
, which is telling the browser that this browser action is now going to display a popup when clicked, the document for which can be found at "popup/choose_page.html".
So now we need to create that popup. Create a directory called "popup" then create a file called "choose_page.html" inside it. Give it the following contents:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="stylesheet" href="choose_page.css" />
</head>
<body>
<div class="page-choice">developer.mozilla.org</div>
<div class="page-choice">support.mozilla.org</div>
<div class="page-choice">addons.mozilla.org</div>
<script src="choose_page.js"></script>
</body>
</html>
You can see that this is a normal HTML page containing three <div>
elements, each with the name of a Mozilla site inside. It also includes a CSS file and a JavaScript file, which we'll add next.
Create a file called "choose_page.css" inside the "popup" directory, and give it these contents:
html,
body {
width: 300px;
}
.page-choice {
width: 100%;
padding: 4px;
font-size: 1.5em;
text-align: center;
cursor: pointer;
}
.page-choice:hover {
background-color: #cff2f2;
}
This is just a bit of styling for our popup.
Next, create a "choose_page.js" file inside the "popup" directory, and give it these contents:
document.addEventListener("click", (event) => {
if (!event.target.classList.contains("page-choice")) {
return;
}
const chosenPage = `https://${event.target.textContent}`;
browser.tabs.create({
url: chosenPage,
});
});
In our JavaScript, we listen for clicks on the popup choices. We first check to see if the click landed on one of the page-choices; if not, we don't do anything else. If the click did land on a page-choice, we construct a URL from it, and open a new tab containing the corresponding page. Note that we can use WebExtension APIs in popup scripts, just as we can in background scripts.
The extension's final structure should look like this:
button/
icons/
page-16.png
page-32.png
popup/
choose_page.css
choose_page.html
choose_page.js
manifest.json
Now reload the extension, click the button again, and try clicking on the choices in the popup:
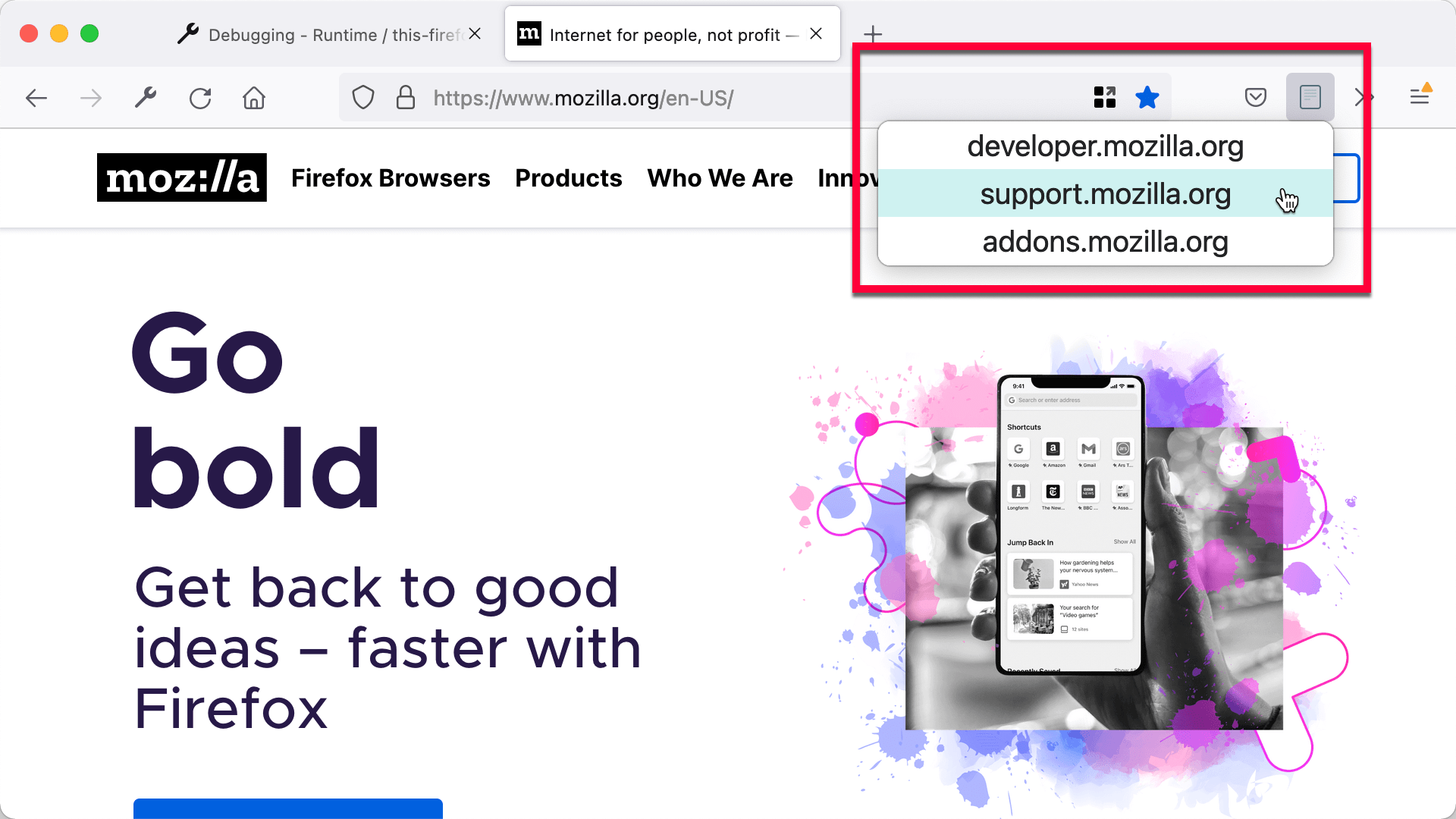