On this page
Web on Servlet Stack
This part of the documentation covers support for Servlet-stack web applications built on the Servlet API and deployed to Servlet containers. Individual chapters include Spring MVC, View Technologies, CORS Support, and WebSocket Support. For reactive-stack web applications, see Web on Reactive Stack.
1. Spring Web MVC
Spring Web MVC is the original web framework built on the Servlet API and has been included in the Spring Framework from the very beginning. The formal name, “Spring Web MVC,” comes from the name of its source module (spring-webmvc
), but it is more commonly known as “Spring MVC”.
Parallel to Spring Web MVC, Spring Framework 5.0 introduced a reactive-stack web framework whose name, “Spring WebFlux,” is also based on its source module (spring-webflux
). This section covers Spring Web MVC. The next section covers Spring WebFlux.
For baseline information and compatibility with Servlet container and Java EE version ranges, see the Spring Framework Wiki .
1.1. DispatcherServlet
Spring MVC, as many other web frameworks, is designed around the front controller pattern where a central Servlet
, the DispatcherServlet
, provides a shared algorithm for request processing, while actual work is performed by configurable delegate components. This model is flexible and supports diverse workflows.
The DispatcherServlet
, as any Servlet
, needs to be declared and mapped according to the Servlet specification by using Java configuration or in web.xml
. In turn, the DispatcherServlet
uses Spring configuration to discover the delegate components it needs for request mapping, view resolution, exception handling, and more.
The following example of the Java configuration registers and initializes the DispatcherServlet
, which is auto-detected by the Servlet container (see Servlet Config):
public class MyWebApplicationInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext servletCxt) {
// Load Spring web application configuration
AnnotationConfigWebApplicationContext ac = new AnnotationConfigWebApplicationContext();
ac.register(AppConfig.class);
ac.refresh();
// Create and register the DispatcherServlet
DispatcherServlet servlet = new DispatcherServlet(ac);
ServletRegistration.Dynamic registration = servletCxt.addServlet("app", servlet);
registration.setLoadOnStartup(1);
registration.addMapping("/app/*");
}
}
In addition to using the ServletContext API directly, you can also extend AbstractAnnotationConfigDispatcherServletInitializer and override specific methods (see the example under Context Hierarchy). |
The following example of web.xml
configuration registers and initializes the DispatcherServlet
:
<web-app>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/app-context.xml</param-value>
</context-param>
<servlet>
<servlet-name>app</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value></param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>app</servlet-name>
<url-pattern>/app/*</url-pattern>
</servlet-mapping>
</web-app>
Spring Boot follows a different initialization sequence. Rather than hooking into the lifecycle of the Servlet container, Spring Boot uses Spring configuration to bootstrap itself and the embedded Servlet container. Filter and Servlet declarations are detected in Spring configuration and registered with the Servlet container. For more details, see the Spring Boot documentation . |
1.1.1. Context Hierarchy
DispatcherServlet
expects a WebApplicationContext
(an extension of a plain ApplicationContext
) for its own configuration. WebApplicationContext
has a link to the ServletContext
and the Servlet
with which it is associated. It is also bound to the ServletContext
such that applications can use static methods on RequestContextUtils
to look up the WebApplicationContext
if they need access to it.
For many applications, having a single WebApplicationContext
is simple and suffices. It is also possible to have a context hierarchy where one root WebApplicationContext
is shared across multiple DispatcherServlet
(or other Servlet
) instances, each with its own child WebApplicationContext
configuration. See Additional Capabilities of the ApplicationContext
for more on the context hierarchy feature.
The root WebApplicationContext
typically contains infrastructure beans, such as data repositories and business services that need to be shared across multiple Servlet
instances. Those beans are effectively inherited and can be overridden (that is, re-declared) in the Servlet-specific child WebApplicationContext
, which typically contains beans local to the given Servlet
. The following image shows this relationship:
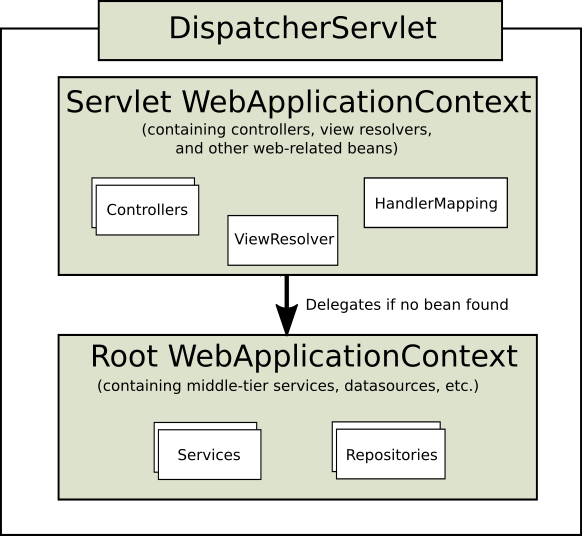
The following example configures a WebApplicationContext
hierarchy:
public class MyWebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return new Class<?>[] { RootConfig.class };
}
@Override
protected Class<?>[] getServletConfigClasses() {
return new Class<?>[] { App1Config.class };
}
@Override
protected String[] getServletMappings() {
return new String[] { "/app1/*" };
}
}
If an application context hierarchy is not required, applications can return all configuration through getRootConfigClasses() and null from getServletConfigClasses() . |
The following example shows the web.xml
equivalent:
<web-app>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/root-context.xml</param-value>
</context-param>
<servlet>
<servlet-name>app1</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/app1-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>app1</servlet-name>
<url-pattern>/app1/*</url-pattern>
</servlet-mapping>
</web-app>
If an application context hierarchy is not required, applications may configure a “root” context only and leave the contextConfigLocation Servlet parameter empty. |
1.1.2. Special Bean Types
The DispatcherServlet
delegates to special beans to process requests and render the appropriate responses. By “special beans” we mean Spring-managed Object
instances that implement WebFlux framework contracts. Those usually come with built-in contracts, but you can customize their properties and extend or replace them.
The following table lists the special beans detected by the DispatcherHandler
:
Bean type | Explanation |
---|---|
|
Map a request to a handler along with a list of interceptors for pre- and post-processing. The mapping is based on some criteria, the details of which vary by The two main |
|
Help the |
Strategy to resolve exceptions, possibly mapping them to handlers, to HTML error views, or other targets. See Exceptions. |
|
Resolve logical |
|
Resolve the |
|
Resolve themes your web application can use — for example, to offer personalized layouts. See Themes. |
|
Abstraction for parsing a multi-part request (for example, browser form file upload) with the help of some multipart parsing library. See Multipart Resolver. |
|
Store and retrieve the “input” and the “output” |
1.1.3. Web MVC Config
Applications can declare the infrastructure beans listed in Special Bean Types that are required to process requests. The DispatcherServlet
checks the WebApplicationContext
for each special bean. If there are no matching bean types, it falls back on the default types listed in DispatcherServlet.properties
.
In most cases, the MVC Config is the best starting point. It declares the required beans in either Java or XML and provides a higher-level configuration callback API to customize it.
Spring Boot relies on the MVC Java configuration to configure Spring MVC and provides many extra convenient options. |
1.1.4. Servlet Config
In a Servlet 3.0+ environment, you have the option of configuring the Servlet container programmatically as an alternative or in combination with a web.xml
file. The following example registers a DispatcherServlet
:
import org.springframework.web.WebApplicationInitializer;
public class MyWebApplicationInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext container) {
XmlWebApplicationContext appContext = new XmlWebApplicationContext();
appContext.setConfigLocation("/WEB-INF/spring/dispatcher-config.xml");
ServletRegistration.Dynamic registration = container.addServlet("dispatcher", new DispatcherServlet(appContext));
registration.setLoadOnStartup(1);
registration.addMapping("/");
}
}
WebApplicationInitializer
is an interface provided by Spring MVC that ensures your implementation is detected and automatically used to initialize any Servlet 3 container. An abstract base class implementation of WebApplicationInitializer
named AbstractDispatcherServletInitializer
makes it even easier to register the DispatcherServlet
by overriding methods to specify the servlet mapping and the location of the DispatcherServlet
configuration.
This is recommended for applications that use Java-based Spring configuration, as the following example shows:
public class MyWebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return null;
}
@Override
protected Class<?>[] getServletConfigClasses() {
return new Class<?>[] { MyWebConfig.class };
}
@Override
protected String[] getServletMappings() {
return new String[] { "/" };
}
}
If you use XML-based Spring configuration, you should extend directly from AbstractDispatcherServletInitializer
, as the following example shows:
public class MyWebAppInitializer extends AbstractDispatcherServletInitializer {
@Override
protected WebApplicationContext createRootApplicationContext() {
return null;
}
@Override
protected WebApplicationContext createServletApplicationContext() {
XmlWebApplicationContext cxt = new XmlWebApplicationContext();
cxt.setConfigLocation("/WEB-INF/spring/dispatcher-config.xml");
return cxt;
}
@Override
protected String[] getServletMappings() {
return new String[] { "/" };
}
}
AbstractDispatcherServletInitializer
also provides a convenient way to add Filter
instances and have them be automatically mapped to the DispatcherServlet
, as the following example shows:
public class MyWebAppInitializer extends AbstractDispatcherServletInitializer {
// ...
@Override
protected Filter[] getServletFilters() {
return new Filter[] {
new HiddenHttpMethodFilter(), new CharacterEncodingFilter() };
}
}
Each filter is added with a default name based on its concrete type and automatically mapped to the DispatcherServlet
.
The isAsyncSupported
protected method of AbstractDispatcherServletInitializer
provides a single place to enable async support on the DispatcherServlet
and all filters mapped to it. By default, this flag is set to true
.
Finally, if you need to further customize the DispatcherServlet
itself, you can override the createDispatcherServlet
method.
1.1.5. Processing
The DispatcherServlet
processes requests as follows:
The
WebApplicationContext
is searched for and bound in the request as an attribute that the controller and other elements in the process can use. It is bound by default under theDispatcherServlet.WEB_APPLICATION_CONTEXT_ATTRIBUTE
key.The locale resolver is bound to the request to let elements in the process resolve the locale to use when processing the request (rendering the view, preparing data, and so on). If you do not need locale resolving, you do not need the locale resolver.
The theme resolver is bound to the request to let elements such as views determine which theme to use. If you do not use themes, you can ignore it.
If you specify a multipart file resolver, the request is inspected for multiparts. If multiparts are found, the request is wrapped in a
MultipartHttpServletRequest
for further processing by other elements in the process. See Multipart Resolver for further information about multipart handling.An appropriate handler is searched for. If a handler is found, the execution chain associated with the handler (preprocessors, postprocessors, and controllers) is executed in order to prepare a model or rendering. Alternatively, for annotated controllers, the response can be rendered (within the
HandlerAdapter
) instead of returning a view.If a model is returned, the view is rendered. If no model is returned (maybe due to a preprocessor or postprocessor intercepting the request, perhaps for security reasons), no view is rendered, because the request could already have been fulfilled.
The HandlerExceptionResolver
beans declared in the WebApplicationContext
are used to resolve exceptions thrown during request processing. Those exception resolvers allow customizing the logic to address exceptions. See Exceptions for more details.
The Spring DispatcherServlet
also supports the return of the last-modification-date
, as specified by the Servlet API. The process of determining the last modification date for a specific request is straightforward: The DispatcherServlet
looks up an appropriate handler mapping and tests whether the handler that is found implements the LastModified
interface. If so, the value of the long getLastModified(request)
method of the LastModified
interface is returned to the client.
You can customize individual DispatcherServlet
instances by adding Servlet initialization parameters (init-param
elements) to the Servlet declaration in the web.xml
file. The following table lists the supported parameters:
Parameter | Explanation |
---|---|
|
Class that implements |
|
String that is passed to the context instance (specified by |
|
Namespace of the |
|
Whether to throw a By default, this is set to Note that, if default servlet handling is also configured, unresolved requests are always forwarded to the default servlet and a 404 is never raised. |
1.1.6. Interception
All HandlerMapping
implementations support handler interceptors that are useful when you want to apply specific functionality to certain requests — for example, checking for a principal. Interceptors must implement HandlerInterceptor
from the org.springframework.web.servlet
package with three methods that should provide enough flexibility to do all kinds of pre-processing and post-processing:
preHandle(..)
: Before the actual handler is executedpostHandle(..)
: After the handler is executedafterCompletion(..)
: After the complete request has finished
The preHandle(..)
method returns a boolean value. You can use this method to break or continue the processing of the execution chain. When this method returns true
, the handler execution chain continues. When it returns false, the DispatcherServlet
assumes the interceptor itself has taken care of requests (and, for example, rendered an appropriate view) and does not continue executing the other interceptors and the actual handler in the execution chain.
See Interceptors in the section on MVC configuration for examples of how to configure interceptors. You can also register them directly by using setters on individual HandlerMapping
implementations.
Note that postHandle
is less useful with @ResponseBody
and ResponseEntity
methods for which the response is written and committed within the HandlerAdapter
and before postHandle
. That means it is too late to make any changes to the response, such as adding an extra header. For such scenarios, you can implement ResponseBodyAdvice
and either declare it as an Controller Advice bean or configure it directly on RequestMappingHandlerAdapter
.
1.1.7. Exceptions
If an exception occurs during request mapping or is thrown from a request handler (such as a @Controller
), the DispatcherServlet
delegates to a chain of HandlerExceptionResolver
beans to resolve the exception and provide alternative handling, which is typically an error response.
The following table lists the available HandlerExceptionResolver
implementations:
HandlerExceptionResolver |
Description |
---|---|
|
A mapping between exception class names and error view names. Useful for rendering error pages in a browser application. |
Resolves exceptions raised by Spring MVC and maps them to HTTP status codes. See also alternative |
|
|
Resolves exceptions with the |
|
Resolves exceptions by invoking an |
Chain of Resolvers
You can form an exception resolver chain by declaring multiple HandlerExceptionResolver
beans in your Spring configuration and setting their order
properties as needed. The higher the order property, the later the exception resolver is positioned.
The contract of HandlerExceptionResolver
specifies that it can return:
a
ModelAndView
that points to an error view.An empty
ModelAndView
if the exception was handled within the resolver.null
if the exception remains unresolved, for subsequent resolvers to try, and, if the exception remains at the end, it is allowed to bubble up to the Servlet container.
The MVC Config automatically declares built-in resolvers for default Spring MVC exceptions, for @ResponseStatus
annotated exceptions, and for support of @ExceptionHandler
methods. You can customize that list or replace it.
Container Error Page
If an exception remains unresolved by any HandlerExceptionResolver
and is, therefore, left to propagate or if the response status is set to an error status (that is, 4xx, 5xx), Servlet containers can render a default error page in HTML. To customize the default error page of the container, you can declare an error page mapping in web.xml
. The following example shows how to do so:
<error-page>
<location>/error</location>
</error-page>
Given the preceding example, when an exception bubbles up or the response has an error status, the Servlet container makes an ERROR dispatch within the container to the configured URL (for example, /error
). This is then processed by the DispatcherServlet
, possibly mapping it to a @Controller
, which could be implemented to return an error view name with a model or to render a JSON response, as the following example shows:
@RestController
public class ErrorController {
@RequestMapping(path = "/error")
public Map<String, Object> handle(HttpServletRequest request) {
Map<String, Object> map = new HashMap<String, Object>();
map.put("status", request.getAttribute("javax.servlet.error.status_code"));
map.put("reason", request.getAttribute("javax.servlet.error.message"));
return map;
}
}
The Servlet API does not provide a way to create error page mappings in Java. You can, however, use both a WebApplicationInitializer and a minimal web.xml . |
1.1.8. View Resolution
Spring MVC defines the ViewResolver
and View
interfaces that let you render models in a browser without tying you to a specific view technology. ViewResolver
provides a mapping between view names and actual views. View
addresses the preparation of data before handing over to a specific view technology.
The following table provides more details on the ViewResolver
hierarchy:
ViewResolver | Description |
---|---|
|
Sub-classes of |
|
Implementation of |
|
Implementation of |
|
Simple implementation of the |
|
Convenient subclass of |
|
Convenient subclass of |
|
Implementation of the |
Handling
You can chain view resolvers by declaring more than one resolver bean and, if necessary, by setting the order
property to specify ordering. Remember, the higher the order property, the later the view resolver is positioned in the chain.
The contract of a ViewResolver
specifies that it can return null to indicate that the view could not be found. However, in the case of JSPs and InternalResourceViewResolver
, the only way to figure out if a JSP exists is to perform a dispatch through RequestDispatcher
. Therefore, you must always configure an InternalResourceViewResolver
to be last in the overall order of view resolvers.
Configuring view resolution is as simple as adding ViewResolver
beans to your Spring configuration. The MVC Config provides a dedicated configuration API for View Resolvers and for adding logic-less View Controllers which are useful for HTML template rendering without controller logic.
Redirecting
The special redirect:
prefix in a view name lets you perform a redirect. The UrlBasedViewResolver
(and its subclasses) recognize this as an instruction that a redirect is needed. The rest of the view name is the redirect URL.
The net effect is the same as if the controller had returned a RedirectView
, but now the controller itself can operate in terms of logical view names. A logical view name (such as redirect:/myapp/some/resource
) redirects relative to the current Servlet context, while a name such as redirect:http://myhost.com/some/arbitrary/path
redirects to an absolute URL.
Note that, if a controller method is annotated with the @ResponseStatus
, the annotation value takes precedence over the response status set by RedirectView
.
Forwarding
You can also use a special forward:
prefix for view names that are ultimately resolved by UrlBasedViewResolver
and subclasses. This creates an InternalResourceView
, which does a RequestDispatcher.forward()
. Therefore, this prefix is not useful with InternalResourceViewResolver
and InternalResourceView
(for JSPs), but it can be helpful if you use another view technology but still want to force a forward of a resource to be handled by the Servlet/JSP engine. Note that you may also chain multiple view resolvers, instead.
Content Negotiation
ContentNegotiatingViewResolver
does not resolve views itself but rather delegates to other view resolvers and selects the view that resembles the representation requested by the client. The representation can be determined from the Accept
header or from a query parameter (for example, "/path?format=pdf"
).
The ContentNegotiatingViewResolver
selects an appropriate View
to handle the request by comparing the request media types with the media type (also known as Content-Type
) supported by the View
associated with each of its ViewResolvers
. The first View
in the list that has a compatible Content-Type
returns the representation to the client. If a compatible view cannot be supplied by the ViewResolver
chain, the list of views specified through the DefaultViews
property is consulted. This latter option is appropriate for singleton Views
that can render an appropriate representation of the current resource regardless of the logical view name. The Accept
header can include wildcards (for example text/*
), in which case a View
whose Content-Type
is text/xml
is a compatible match.
See View Resolvers under MVC Config for configuration details.
1.1.9. Locale
Most parts of Spring’s architecture support internationalization, as the Spring web MVC framework does. DispatcherServlet
lets you automatically resolve messages by using the client’s locale. This is done with LocaleResolver
objects.
When a request comes in, the DispatcherServlet
looks for a locale resolver and, if it finds one, it tries to use it to set the locale. By using the RequestContext.getLocale()
method, you can always retrieve the locale that was resolved by the locale resolver.
In addition to automatic locale resolution, you can also attach an interceptor to the handler mapping (see Interception for more information on handler mapping interceptors) to change the locale under specific circumstances (for example, based on a parameter in the request).
Locale resolvers and interceptors are defined in the org.springframework.web.servlet.i18n
package and are configured in your application context in the normal way. The following selection of locale resolvers is included in Spring.
Time Zone
In addition to obtaining the client’s locale, it is often useful to know its time zone. The LocaleContextResolver
interface offers an extension to LocaleResolver
that lets resolvers provide a richer LocaleContext
, which may include time zone information.
When available, the user’s TimeZone
can be obtained by using the RequestContext.getTimeZone()
method. Time zone information is automatically used by any Date/Time Converter
and Formatter
objects that are registered with Spring’s ConversionService
.
Header Resolver
This locale resolver inspects the accept-language
header in the request that was sent by the client (for example, a web browser). Usually, this header field contains the locale of the client’s operating system. Note that this resolver does not support time zone information.
Cookie Resolver
This locale resolver inspects a Cookie
that might exist on the client to see if a Locale
or TimeZone
is specified. If so, it uses the specified details. By using the properties of this locale resolver, you can specify the name of the cookie as well as the maximum age. The following example defines a CookieLocaleResolver
:
<bean id="localeResolver" class="org.springframework.web.servlet.i18n.CookieLocaleResolver">
<property name="cookieName" value="clientlanguage"/>
<!-- in seconds. If set to -1, the cookie is not persisted (deleted when browser shuts down) -->
<property name="cookieMaxAge" value="100000"/>
</bean>
The following table describes the properties CookieLocaleResolver
:
Property | Default | Description |
---|---|---|
|
classname + LOCALE |
The name of the cookie |
|
Servlet container default |
The maximum time a cookie persists on the client. If |
|
/ |
Limits the visibility of the cookie to a certain part of your site. When |
Session Resolver
The SessionLocaleResolver
lets you retrieve Locale
and TimeZone
from the session that might be associated with the user’s request. In contrast to CookieLocaleResolver
, this strategy stores locally chosen locale settings in the Servlet container’s HttpSession
. As a consequence, those settings are temporary for each session and are, therefore, lost when each session terminates.
Note that there is no direct relationship with external session management mechanisms, such as the Spring Session project. This SessionLocaleResolver
evaluates and modifies the corresponding HttpSession
attributes against the current HttpServletRequest
.
Locale Interceptor
You can enable changing of locales by adding the LocaleChangeInterceptor
to one of the HandlerMapping
definitions. It detects a parameter in the request and changes the locale accordingly, calling the setLocale
method on the LocaleResolver
in the dispatcher’s application context. The next example shows that calls to all *.view
resources that contain a parameter named siteLanguage
now changes the locale. So, for example, a request for the URL, http://www.sf.net/home.view?siteLanguage=nl
, changes the site language to Dutch. The following example shows how to intercept the locale:
<bean id="localeChangeInterceptor"
class="org.springframework.web.servlet.i18n.LocaleChangeInterceptor">
<property name="paramName" value="siteLanguage"/>
</bean>
<bean id="localeResolver"
class="org.springframework.web.servlet.i18n.CookieLocaleResolver"/>
<bean id="urlMapping"
class="org.springframework.web.servlet.handler.SimpleUrlHandlerMapping">
<property name="interceptors">
<list>
<ref bean="localeChangeInterceptor"/>
</list>
</property>
<property name="mappings">
<value>/**/*.view=someController</value>
</property>
</bean>
1.1.10. Themes
You can apply Spring Web MVC framework themes to set the overall look-and-feel of your application, thereby enhancing user experience. A theme is a collection of static resources, typically style sheets and images, that affect the visual style of the application.
Defining a theme
To use themes in your web application, you must set up an implementation of the org.springframework.ui.context.ThemeSource
interface. The WebApplicationContext
interface extends ThemeSource
but delegates its responsibilities to a dedicated implementation. By default, the delegate is an org.springframework.ui.context.support.ResourceBundleThemeSource
implementation that loads properties files from the root of the classpath. To use a custom ThemeSource
implementation or to configure the base name prefix of the ResourceBundleThemeSource
, you can register a bean in the application context with the reserved name, themeSource
. The web application context automatically detects a bean with that name and uses it.
When you use the ResourceBundleThemeSource
, a theme is defined in a simple properties file. The properties file lists the resources that make up the theme, as the following example shows:
styleSheet=/themes/cool/style.css
background=/themes/cool/img/coolBg.jpg
The keys of the properties are the names that refer to the themed elements from view code. For a JSP, you typically do this using the spring:theme
custom tag, which is very similar to the spring:message
tag. The following JSP fragment uses the theme defined in the previous example to customize the look and feel:
<%@ taglib prefix="spring" uri="http://www.springframework.org/tags"%>
<html>
<head>
<link rel="stylesheet" href="<spring:theme code='styleSheet'/>" type="text/css"/>
</head>
<body style="background=<spring:theme code='background'/>">
...
</body>
</html>
By default, the ResourceBundleThemeSource
uses an empty base name prefix. As a result, the properties files are loaded from the root of the classpath. Thus, you would put the cool.properties
theme definition in a directory at the root of the classpath (for example, in /WEB-INF/classes
). The ResourceBundleThemeSource
uses the standard Java resource bundle loading mechanism, allowing for full internationalization of themes. For example, we could have a /WEB-INF/classes/cool_nl.properties
that references a special background image with Dutch text on it.
Resolving Themes
After you define themes, as described in the preceding section, you decide which theme to use. The DispatcherServlet
looks for a bean named themeResolver
to find out which ThemeResolver
implementation to use. A theme resolver works in much the same way as a LocaleResolver
. It detects the theme to use for a particular request and can also alter the request’s theme. The following table describes the theme resolvers provided by Spring:
Class | Description |
---|---|
|
Selects a fixed theme, set by using the |
|
The theme is maintained in the user’s HTTP session. It needs to be set only once for each session but is not persisted between sessions. |
|
The selected theme is stored in a cookie on the client. |
Spring also provides a ThemeChangeInterceptor
that lets theme changes on every request with a simple request parameter.
1.1.11. Multipart Resolver
MultipartResolver
from the org.springframework.web.multipart
package is a strategy for parsing multipart requests including file uploads. There is one implementation based on Commons FileUpload and another based on Servlet 3.0 multipart request parsing.
To enable multipart handling, you need to declare a MultipartResolver
bean in your DispatcherServlet
Spring configuration with a name of multipartResolver
. The DispatcherServlet
detects it and applies it to the incoming request. When a POST with content-type of multipart/form-data
is received, the resolver parses the content and wraps the current HttpServletRequest
as MultipartHttpServletRequest
to provide access to resolved parts in addition to exposing them as request parameters.
Apache Commons FileUpload
To use Apache Commons FileUpload
, you can configure a bean of type CommonsMultipartResolver
with a name of multipartResolver
. You also need to have commons-fileupload
as a dependency on your classpath.
Servlet 3.0
Servlet 3.0 multipart parsing needs to be enabled through Servlet container configuration. To do so:
In Java, set a
MultipartConfigElement
on the Servlet registration.In
web.xml
, add a"<multipart-config>"
section to the servlet declaration.
The following example shows how to set a MultipartConfigElement
on the Servlet registration:
public class AppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
// ...
@Override
protected void customizeRegistration(ServletRegistration.Dynamic registration) {
// Optionally also set maxFileSize, maxRequestSize, fileSizeThreshold
registration.setMultipartConfig(new MultipartConfigElement("/tmp"));
}
}
Once the Servlet 3.0 configuration is in place, you can add a bean of type StandardServletMultipartResolver
with a name of multipartResolver
.
1.1.12. Logging
DEBUG-level logging in Spring MVC is designed to be compact, minimal, and human-friendly. It focuses on high-value bits of information that are useful over and over again versus others that are useful only when debugging a specific issue.
TRACE-level logging generally follows the same principles as DEBUG (and, for example, also should not be a fire hose) but can be used for debugging any issue. In addition, some log messages may show a different level of detail at TRACE versus DEBUG.
Good logging comes from the experience of using the logs. If you spot anything that does not meet the stated goals, please let us know.
Sensitive Data
DEBUG and TRACE logging may log sensitive information. This is why request parameters and headers are masked by default and their logging in full must be enabled explicitly through the enableLoggingRequestDetails
property on DispatcherServlet
.
The following example shows how to do so by using Java configuration:
public class MyInitializer
extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return ... ;
}
@Override
protected Class<?>[] getServletConfigClasses() {
return ... ;
}
@Override
protected String[] getServletMappings() {
return ... ;
}
@Override
protected void customizeRegistration(Dynamic registration) {
registration.setInitParameter("enableLoggingRequestDetails", "true");
}
}
1.2. Filters
The spring-web
module provides some useful filters:
1.2.1. Form Data
Browsers can submit form data only through HTTP GET or HTTP POST but non-browser clients can also use HTTP PUT, PATCH, and DELETE. The Servlet API requires ServletRequest.getParameter*()
methods to support form field access only for HTTP POST.
The spring-web
module provides FormContentFilter
to intercept HTTP PUT, PATCH, and DELETE requests with a content type of application/x-www-form-urlencoded
, read the form data from the body of the request, and wrap the ServletRequest
to make the form data available through the ServletRequest.getParameter*()
family of methods.
1.2.2. Forwarded Headers
As a request goes through proxies (such as load balancers) the host, port, and scheme may change, and that makes it a challenge to create links that point to the correct host, port, and scheme from a client perspective.
RFC 7239 defines the Forwarded
HTTP header that proxies can use to provide information about the original request. There are other non-standard headers, too, including X-Forwarded-Host
, X-Forwarded-Port
, X-Forwarded-Proto
, X-Forwarded-Ssl
, and X-Forwarded-Prefix
.
ForwardedHeaderFilter
is a Servlet filter that modifies the host, port, and scheme of the request, based on Forwarded
headers, and then removes those headers.
There are security considerations for forwarded headers since an application cannot know if the headers were added by a proxy, as intended, or by a malicious client. This is why a proxy at the boundary of trust should be configured to remove untrusted Forwarded
headers that come from the outside. You can also configure the ForwardedHeaderFilter
with removeOnly=true
, in which case it removes but does not use the headers.
1.2.3. Shallow ETag
The ShallowEtagHeaderFilter
filter creates a “shallow” ETag by caching the content written to the response and computing an MD5 hash from it. The next time a client sends, it does the same, but it also compares the computed value against the If-None-Match
request header and, if the two are equal, returns a 304 (NOT_MODIFIED).
This strategy saves network bandwidth but not CPU, as the full response must be computed for each request. Other strategies at the controller level, described earlier, can avoid the computation. See HTTP Caching.
This filter has a writeWeakETag
parameter that configures the filter to write weak ETags similar to the following: W/"02a2d595e6ed9a0b24f027f2b63b134d6"
(as defined in RFC 7232 Section 2.3 ).
1.2.4. CORS
Spring MVC provides fine-grained support for CORS configuration through annotations on controllers. However, when used with Spring Security, we advise relying on the built-in CorsFilter
that must be ordered ahead of Spring Security’s chain of filters.
See the sections on CORS and the CORS Filter for more details.
1.3. Annotated Controllers
Spring MVC provides an annotation-based programming model where @Controller
and @RestController
components use annotations to express request mappings, request input, exception handling, and more. Annotated controllers have flexible method signatures and do not have to extend base classes nor implement specific interfaces. The following example shows a controller defined by annotations:
@Controller
public class HelloController {
@GetMapping("/hello")
public String handle(Model model) {
model.addAttribute("message", "Hello World!");
return "index";
}
}
In the preceding example, the method accepts a Model
and returns a view name as a String
, but many other options exist and are explained later in this chapter.
Guides and tutorials on spring.io use the annotation-based programming model described in this section. |
1.3.1. Declaration
You can define controller beans by using a standard Spring bean definition in the Servlet’s WebApplicationContext
. The @Controller
stereotype allows for auto-detection, aligned with Spring general support for detecting @Component
classes in the classpath and auto-registering bean definitions for them. It also acts as a stereotype for the annotated class, indicating its role as a web component.
To enable auto-detection of such @Controller
beans, you can add component scanning to your Java configuration, as the following example shows:
@Configuration
@ComponentScan("org.example.web")
public class WebConfig {
// ...
}
The following example shows the XML configuration equivalent of the preceding example:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:component-scan base-package="org.example.web"/>
<!-- ... -->
</beans>
@RestController
is a composed annotation that is itself meta-annotated with @Controller
and @ResponseBody
to indicate a controller whose every method inherits the type-level @ResponseBody
annotation and, therefore, writes directly to the response body versus view resolution and rendering with an HTML template.
AOP Proxies
In some cases, you many need to decorate a controller with an AOP proxy at runtime. One example is if you choose to have @Transactional
annotations directly on the controller. When this is the case, for controllers specifically, we recommend using class-based proxying. This is typically the default choice with controllers. However, if a controller must implement an interface that is not a Spring Context callback (such as InitializingBean
, *Aware
, and others), you may need to explicitly configure class-based proxying. For example, with <tx:annotation-driven/>
, you can change to <tx:annotation-driven proxy-target-class="true"/>
.
1.3.2. Request Mapping
You can use the @RequestMapping
annotation to map requests to controllers methods. It has various attributes to match by URL, HTTP method, request parameters, headers, and media types. You can use it at the class level to express shared mappings or at the method level to narrow down to a specific endpoint mapping.
There are also HTTP method specific shortcut variants of @RequestMapping
:
@GetMapping
@PostMapping
@PutMapping
@DeleteMapping
@PatchMapping
The shortcuts are Custom Annotations that are provided because, arguably, most controller methods should be mapped to a specific HTTP method versus using @RequestMapping
, which, by default, matches to all HTTP methods. At the same, a @RequestMapping
is still needed at the class level to express shared mappings.
The following example has type and method level mappings:
@RestController
@RequestMapping("/persons")
class PersonController {
@GetMapping("/{id}")
public Person getPerson(@PathVariable Long id) {
// ...
}
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
public void add(@RequestBody Person person) {
// ...
}
}
URI patterns
You can map requests by using the following glob patterns and wildcards:
?
matches one character*
matches zero or more characters within a path segment**
match zero or more path segments
You can also declare URI variables and access their values with @PathVariable
, as the following example shows:
@GetMapping("/owners/{ownerId}/pets/{petId}")
public Pet findPet(@PathVariable Long ownerId, @PathVariable Long petId) {
// ...
}
You can declare URI variables at the class and method levels, as the following example shows:
@Controller
@RequestMapping("/owners/{ownerId}")
public class OwnerController {
@GetMapping("/pets/{petId}")
public Pet findPet(@PathVariable Long ownerId, @PathVariable Long petId) {
// ...
}
}
URI variables are automatically converted to the appropriate type, or TypeMismatchException
is raised. Simple types (int
, long
, Date
, and so on) are supported by default and you can register support for any other data type. See Type Conversion and DataBinder
.
You can explicitly name URI variables (for example, @PathVariable("customId")
), but you can leave that detail out if the names are the same and your code is compiled with debugging information or with the -parameters
compiler flag on Java 8.
The syntax {varName:regex}
declares a URI variable with a regular expression that has syntax of {varName:regex}
. For example, given URL "/spring-web-3.0.5 .jar"
, the following method extracts the name, version, and file extension:
@GetMapping("/{name:[a-z-]+}-{version:\\d\\.\\d\\.\\d}{ext:\\.[a-z]+}")
public void handle(@PathVariable String version, @PathVariable String ext) {
// ...
}
URI path patterns can also have embedded ${…}
placeholders that are resolved on startup by using PropertyPlaceHolderConfigurer
against local, system, environment, and other property sources. You can use this, for example, to parameterize a base URL based on some external configuration.
Spring MVC uses the PathMatcher contract and the AntPathMatcher implementation from spring-core for URI path matching. |
Pattern Comparison
When multiple patterns match a URL, they must be compared to find the best match. This is done by using AntPathMatcher.getPatternComparator(String path)
, which looks for patterns that are more specific.
A pattern is less specific if it has a lower count of URI variables and single wildcards counted as 1 and double wildcards counted as 2. Given an equal score, the longer pattern is chosen. Given the same score and length, the pattern with more URI variables than wildcards is chosen.
The default mapping pattern (/**
) is excluded from scoring and always sorted last. Also, prefix patterns (such as /public/**
) are considered less specific than other pattern that do not have double wildcards.
For the full details, see AntPatternComparator
in AntPathMatcher
and also keep mind that you can customize the PathMatcher
implementation. See Path Matching in the configuration section.
Suffix Match
By default, Spring MVC performs .*
suffix pattern matching so that a controller mapped to /person
is also implicitly mapped to /person.*
. The file extension is then used to interpret the requested content type to use for the response (that is, instead of the Accept
header) — for example, /person.pdf
, /person.xml
, and others.
Using file extensions in this way was necessary when browsers used to send Accept
headers that were hard to interpret consistently. At present, that is no longer a necessity and using the Accept
header should be the preferred choice.
Over time, the use of file name extensions has proven problematic in a variety of ways. It can cause ambiguity when overlain with the use of URI variables, path parameters, and URI encoding. Reasoning about URL-based authorization and security (see next section for more details) also become more difficult.
To completely disable the use of file extensions, you must set both of the following:
useSuffixPatternMatching(false)
, see PathMatchConfigurerfavorPathExtension(false)
, see ContentNegotiationConfigurer
URL-based content negotiation can still be useful (for example, when typing a URL in a browser). To enable that, we recommend a query parameter-based strategy to avoid most of the issues that come with file extensions. Alternatively, if you must use file extensions, consider restricting them to a list of explicitly registered extensions through the mediaTypes
property of ContentNegotiationConfigurer.
Suffix Match and RFD
A reflected file download (RFD) attack is similar to XSS in that it relies on request input (for example, a query parameter and a URI variable) being reflected in the response. However, instead of inserting JavaScript into HTML, an RFD attack relies on the browser switching to perform a download and treating the response as an executable script when double-clicked later.
In Spring MVC, @ResponseBody
and ResponseEntity
methods are at risk, because they can render different content types, which clients can request through URL path extensions. Disabling suffix pattern matching and using path extensions for content negotiation lower the risk but are not sufficient to prevent RFD attacks.
To prevent RFD attacks, prior to rendering the response body, Spring MVC adds a Content-Disposition:inline;filename=f.txt
header to suggest a fixed and safe download file. This is done only if the URL path contains a file extension that is neither whitelisted nor explicitly registered for content negotiation. However, it can potentially have side effects when URLs are typed directly into a browser.
Many common path extensions are whitelisted by default. Applications with custom HttpMessageConverter
implementations can explicitly register file extensions for content negotiation to avoid having a Content-Disposition
header added for those extensions. See Content Types.
See CVE-2015-5211 for additional recommendations related to RFD.
Consumable Media Types
You can narrow the request mapping based on the Content-Type
of the request, as the following example shows:
@PostMapping(path = "/pets", consumes = "application/json") (1)
public void addPet(@RequestBody Pet pet) {
// ...
}
1 | Using a consumes attribute to narrow the mapping by the content type. |
The consumes
attribute also supports negation expressions — for example, !text/plain
means any content type other than text/plain
.
You can declare a shared consumes
attribute at the class level. Unlike most other request-mapping attributes, however, when used at the class level, a method-level consumes
attribute overrides rather than extends the class-level declaration.
MediaType provides constants for commonly used media types, such as APPLICATION_JSON_VALUE and APPLICATION_XML_VALUE . |
Producible Media Types
You can narrow the request mapping based on the Accept
request header and the list of content types that a controller method produces, as the following example shows:
@GetMapping(path = "/pets/{petId}", produces = "application/json;charset=UTF-8") (1)
@ResponseBody
public Pet getPet(@PathVariable String petId) {
// ...
}
1 | Using a produces attribute to narrow the mapping by the content type. |
The media type can specify a character set. Negated expressions are supported — for example, !text/plain
means any content type other than "text/plain".
For the JSON content type, the UTF-8 charset should be specified even if RFC7159 clearly states that “no charset parameter is defined for this registration”, because some browsers require it to correctly interpret UTF-8 special characters. |
You can declare a shared produces
attribute at the class level. Unlike most other request-mapping attributes, however, when used at the class level, a method-level produces
attribute overrides rather than extends the class-level declaration.
MediaType provides constants for commonly used media types, such as APPLICATION_JSON_UTF8_VALUE and APPLICATION_XML_VALUE . |
Parameters, headers
You can narrow request mappings based on request parameter conditions. You can test for the presence of a request parameter (myParam
), for the absence of one (!myParam
), or for a specific value (myParam=myValue
). The following example shows how to test for a specific value:
@GetMapping(path = "/pets/{petId}", params = "myParam=myValue") (1)
public void findPet(@PathVariable String petId) {
// ...
}
1 | Testing whether myParam equals myValue . |
You can also use the same with request header conditions, as the following example shows:
@GetMapping(path = "/pets", headers = "myHeader=myValue") (1)
public void findPet(@PathVariable String petId) {
// ...
}
1 | Testing whether myHeader equals myValue . |
HTTP HEAD, OPTIONS
@GetMapping
(and @RequestMapping(method=HttpMethod.GET)
) support HTTP HEAD transparently for request mapping. Controller methods do not need to change. A response wrapper, applied in javax.servlet.http.HttpServlet
, ensures a Content-Length
header is set to the number of bytes written (without actually writing to the response).
@GetMapping
(and @RequestMapping(method=HttpMethod.GET)
) are implicitly mapped to and support HTTP HEAD. An HTTP HEAD request is processed as if it were HTTP GET except that, instead of writing the body, the number of bytes are counted and the Content-Length
header is set.
By default, HTTP OPTIONS is handled by setting the Allow
response header to the list of HTTP methods listed in all @RequestMapping
methods that have matching URL patterns.
For a @RequestMapping
without HTTP method declarations, the Allow
header is set to GET,HEAD,POST,PUT,PATCH,DELETE,OPTIONS
. Controller methods should always declare the supported HTTP methods (for example, by using the HTTP method specific variants: @GetMapping
, @PostMapping
, and others).
You can explicitly map the @RequestMapping
method to HTTP HEAD and HTTP OPTIONS, but that is not necessary in the common case.
Custom Annotations
Spring MVC supports the use of composed annotations for request mapping. Those are annotations that are themselves meta-annotated with @RequestMapping
and composed to redeclare a subset (or all) of the @RequestMapping
attributes with a narrower, more specific purpose.
@GetMapping
, @PostMapping
, @PutMapping
, @DeleteMapping
, and @PatchMapping
are examples of composed annotations. They are provided because, arguably, most controller methods should be mapped to a specific HTTP method versus using @RequestMapping
, which, by default, matches to all HTTP methods. If you need an example of composed annotations, look at how those are declared.
Spring MVC also supports custom request-mapping attributes with custom request-matching logic. This is a more advanced option that requires subclassing RequestMappingHandlerMapping
and overriding the getCustomMethodCondition
method, where you can check the custom attribute and return your own RequestCondition
.
Explicit Registrations
You can programmatically register handler methods, which you can use for dynamic registrations or for advanced cases, such as different instances of the same handler under different URLs. The following example registers a handler method:
@Configuration
public class MyConfig {
@Autowired
public void setHandlerMapping(RequestMappingHandlerMapping mapping, UserHandler handler) (1)
throws NoSuchMethodException {
RequestMappingInfo info = RequestMappingInfo
.paths("/user/{id}").methods(RequestMethod.GET).build(); (2)
Method method = UserHandler.class.getMethod("getUser", Long.class); (3)
mapping.registerMapping(info, handler, method); (4)
}
}
1 | Inject the target handler and the handler mapping for controllers. |
2 | Prepare the request mapping meta data. |
3 | Get the handler method. |
4 | Add the registration. |
1.3.3. Handler Methods
@RequestMapping
handler methods have a flexible signature and can choose from a range of supported controller method arguments and return values.
Method Arguments
The next table describes the supported controller method arguments. Reactive types are not supported for any arguments.
JDK 8’s java.util.Optional
is supported as a method argument in combination with annotations that have a required
attribute (for example, @RequestParam
, @RequestHeader
, and others) and is equivalent to required=false
.
Controller method argument | Description |
---|---|
|
Generic access to request parameters and request and session attributes, without direct use of the Servlet API. |
|
Choose any specific request or response type — for example, |
|
Enforces the presence of a session. As a consequence, such an argument is never |
|
Servlet 4.0 push builder API for programmatic HTTP/2 resource pushes. Note that, per the Servlet specification, the injected |
|
Currently authenticated user — possibly a specific |
|
The HTTP method of the request. |
|
The current request locale, determined by the most specific |
|
The time zone associated with the current request, as determined by a |
|
For access to the raw request body as exposed by the Servlet API. |
|
For access to the raw response body as exposed by the Servlet API. |
|
For access to URI template variables. See URI patterns. |
|
For access to name-value pairs in URI path segments. See Matrix Variables. |
|
For access to the Servlet request parameters, including multipart files. Parameter values are converted to the declared method argument type. See Note that use of |
|
For access to request headers. Header values are converted to the declared method argument type. See |
|
For access to cookies. Cookies values are converted to the declared method argument type. See |
|
For access to the HTTP request body. Body content is converted to the declared method argument type by using |
|
For access to request headers and body. The body is converted with an |
|
For access to a part in a |
|
For access to the model that is used in HTML controllers and exposed to templates as part of view rendering. |
|
Specify attributes to use in case of a redirect (that is, to be appended to the query string) and flash attributes to be stored temporarily until the request after redirect. See Redirect Attributes and Flash Attributes. |
|
For access to an existing attribute in the model (instantiated if not present) with data binding and validation applied. See Note that use of |
|
For access to errors from validation and data binding for a command object (that is, a |
|
For marking form processing complete, which triggers cleanup of session attributes declared through a class-level |
|
For preparing a URL relative to the current request’s host, port, scheme, context path, and the literal part of the servlet mapping. See URI Links. |
|
For access to any session attribute, in contrast to model attributes stored in the session as a result of a class-level |
|
For access to request attributes. See |
Any other argument |
If a method argument is not matched to any of the earlier values in this table and it is a simple type (as determined by BeanUtils#isSimpleProperty , it is a resolved as a |
Return Values
The next table describes the supported controller method return values. Reactive types are supported for all return values.
Controller method return value | Description |
---|---|
|
The return value is converted through |
|
The return value that specifies the full response (including HTTP headers and body) is to be converted through |
|
For returning a response with headers and no body. |
|
A view name to be resolved with |
|
A |
|
Attributes to be added to the implicit model, with the view name implicitly determined through a |
|
An attribute to be added to the model, with the view name implicitly determined through a Note that |
|
The view and model attributes to use and, optionally, a response status. |
|
A method with a If none of the above is true, a |
|
Produce any of the preceding return values asynchronously from any thread — for example, as a result of some event or callback. See Asynchronous Requests and |
|
Produce any of the above return values asynchronously in a Spring MVC-managed thread. See Asynchronous Requests and |
|
Alternative to |
|
Emit a stream of objects asynchronously to be written to the response with |
|
Write to the response |
Reactive types — Reactor, RxJava, or others through |
Alternative to For streaming scenarios (for example, See Asynchronous Requests and Reactive Types. |
Any other return value |
Any return value that does not match any of the earlier values in this table and that is a |
Type Conversion
Some annotated controller method arguments that represent String
-based request input (such as @RequestParam
, @RequestHeader
, @PathVariable
, @MatrixVariable
, and @CookieValue
) can require type conversion if the argument is declared as something other than String
.
For such cases, type conversion is automatically applied based on the configured converters. By default, simple types (int
, long
, Date
, and others) are supported. You can customize type conversion through a WebDataBinder
(see DataBinder
) or by registering Formatters
with the FormattingConversionService
. See Spring Field Formatting.
Matrix Variables
RFC 3986 discusses name-value pairs in path segments. In Spring MVC, we refer to those as “matrix variables” based on an “old post” by Tim Berners-Lee, but they can be also be referred to as URI path parameters.
Matrix variables can appear in any path segment, with each variable separated by a semicolon and multiple values separated by comma (for example, /cars;color=red,green;year=2012
). Multiple values can also be specified through repeated variable names (for example, color=red;color=green;color=blue
).
If a URL is expected to contain matrix variables, the request mapping for a controller method must use a URI variable to mask that variable content and ensure the request can be matched successfully independent of matrix variable order and presence. The following example uses a matrix variable:
// GET /pets/42;q=11;r=22
@GetMapping("/pets/{petId}")
public void findPet(@PathVariable String petId, @MatrixVariable int q) {
// petId == 42
// q == 11
}
Given that all path segments may contain matrix variables, you may sometimes need to disambiguate which path variable the matrix variable is expected to be in. The following example shows how to do so:
// GET /owners/42;q=11/pets/21;q=22
@GetMapping("/owners/{ownerId}/pets/{petId}")
public void findPet(
@MatrixVariable(name="q", pathVar="ownerId") int q1,
@MatrixVariable(name="q", pathVar="petId") int q2) {
// q1 == 11
// q2 == 22
}
A matrix variable may be defined as optional and a default value specified, as the following example shows:
// GET /pets/42
@GetMapping("/pets/{petId}")
public void findPet(@MatrixVariable(required=false, defaultValue="1") int q) {
// q == 1
}
To get all matrix variables, you can use a MultiValueMap
, as the following example shows:
// GET /owners/42;q=11;r=12/pets/21;q=22;s=23
@GetMapping("/owners/{ownerId}/pets/{petId}")
public void findPet(
@MatrixVariable MultiValueMap<String, String> matrixVars,
@MatrixVariable(pathVar="petId") MultiValueMap<String, String> petMatrixVars) {
// matrixVars: ["q" : [11,22], "r" : 12, "s" : 23]
// petMatrixVars: ["q" : 22, "s" : 23]
}
Note that you need to enable the use of matrix variables. In the MVC Java configuration, you need to set a UrlPathHelper
with removeSemicolonContent=false
through Path Matching. In the MVC XML namespace, you can set <mvc:annotation-driven enable-matrix-variables="true"/>
.
@RequestParam
You can use the @RequestParam
annotation to bind Servlet request parameters (that is, query parameters or form data) to a method argument in a controller.
The following example shows how to do so:
@Controller
@RequestMapping("/pets")
public class EditPetForm {
// ...
@GetMapping
public String setupForm(@RequestParam("petId") int petId, Model model) { (1)
Pet pet = this.clinic.loadPet(petId);
model.addAttribute("pet", pet);
return "petForm";
}
// ...
}
1 | Using @RequestParam to bind petId . |
By default, method parameters that use this annotation are required, but you can specify that a method parameter is optional by setting the @RequestParam
annotation’s required
flag to false
or by declaring the argument with an java.util.Optional
wrapper.
Type conversion is automatically applied if the target method parameter type is not String
. See Type Conversion.
Declaring the argument type as an array or list allows for resolving multiple parameter values for the same parameter name.
When an @RequestParam
annotation is declared as a Map<String, String>
or MultiValueMap<String, String>
, without a parameter name specified in the annotation, then the map is populated with the request parameter values for each given parameter name.
Note that use of @RequestParam
is optional (for example, to set its attributes). By default, any argument that is a simple value type (as determined by BeanUtils#isSimpleProperty ) and is not resolved by any other argument resolver, is treated as if it were annotated with @RequestParam
.
@RequestHeader
You can use the @RequestHeader
annotation to bind a request header to a method argument in a controller.
Consider the following request, with headers:
Host localhost:8080
Accept text/html,application/xhtml+xml,application/xml;q=0.9
Accept-Language fr,en-gb;q=0.7,en;q=0.3
Accept-Encoding gzip,deflate
Accept-Charset ISO-8859-1,utf-8;q=0.7,*;q=0.7
Keep-Alive 300
The following example gets the value of the Accept-Encoding
and Keep-Alive
headers:
@GetMapping("/demo")
public void handle(
@RequestHeader("Accept-Encoding") String encoding, (1)
@RequestHeader("Keep-Alive") long keepAlive) { (2)
//...
}
1 | Get the value of the Accept-Encoding header. |
2 | Get the value of the Keep-Alive header. |
If the target method parameter type is not String
, type conversion is automatically applied. See Type Conversion.
When an @RequestHeader
annotation is used on a Map<String, String>
, MultiValueMap<String, String>
, or HttpHeaders
argument, the map is populated with all header values.
Built-in support is available for converting a comma-separated string into an array or collection of strings or other types known to the type conversion system. For example, a method parameter annotated with @RequestHeader("Accept") can be of type String but also String[] or List<String> . |
@CookieValue
You can use the @CookieValue
annotation to bind the value of an HTTP cookie to a method argument in a controller.
Consider a request with the following cookie:
JSESSIONID=415A4AC178C59DACE0B2C9CA727CDD84
The following example shows how to get the cookie value:
@GetMapping("/demo")
public void handle(@CookieValue("JSESSIONID") String cookie) { (1)
//...
}
1 | Get the value of the JSESSIONID cookie. |
if the target method parameter type is not String
, type conversion is applied automatically. See Type Conversion.
@ModelAttribute
You can use the @ModelAttribute
annotation on a method argument to access an attribute from the model or have it be instantiated if not present. The model attribute is also overlain with values from HTTP Servlet request parameters whose names match to field names. This is referred to as data binding, and it saves you from having to deal with parsing and converting individual query parameters and form fields. The following example shows how to do so:
@PostMapping("/owners/{ownerId}/pets/{petId}/edit")
public String processSubmit(@ModelAttribute Pet pet) { } (1)
1 | Bind an instance of Pet . |
The Pet
instance above is resolved as follows: