On this page
Insert Documents
This page provides examples of insert operations in MongoDB.
Creating a Collection
If the collection does not currently exist, insert operations will create the collection.
Insert a Single Document
New in version 3.2.
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
- PHP
- Motor
- Java (Async)
- C#
- Perl
- Ruby
- Scala
db.collection.insertOne()
inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, MongoDB adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
To insert a single document using MongoDB Compass :
Navigate to the collection you wish to insert the document into:
- In the left-hand MongoDB Compass navigation pane, click the database to which your target collection belongs.
- From the database view, click the target collection name.
Click the Insert Document button:
For each field in the document, select the field type and fill in the field name and value. Add fields by clicking the last line number, then clicking Add Field After …
- For
Object
types, add nested fields by clicking the last field’s number and selecting Add Field After … - For
Array
types, add additional elements to the array by clicking the last element’s line number and selecting Add Array Element After …
- For
Once all fields have been filled out, click Insert.
The following example inserts a new document into the test.inventory
collection:
pymongo.collection.Collection.insert_one()
inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the PyMongo driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
com.mongodb.client.MongoCollection.insertOne inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
Collection.insertOne() inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the Node.js driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
MongoDB\Collection::insertOne()
inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the PHP driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
motor.motor_asyncio.AsyncIOMotorCollection.insert_one()
inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the Motor driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
com.mongodb.reactivestreams.client.MongoCollection.insertOne inserts a single document into a collection with the Java Reactive Streams Driver :
{ item: "canvas", qty: 100, tags: ["cotton"], size: { h: 28, w: 35.5, uom: "cm" } }
The following example inserts the document above into the inventory
collection. If the document does not specify an _id
field, the driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
IMongoCollection.InsertOne() inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the C# driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
MongoDB::Collection::insert_one() inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the Perl driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
Mongo::Collection#insert_one() inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the Ruby driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
collection.insertOne() inserts a single document into a collection.
The following example inserts a new document into the inventory
collection. If the document does not specify an _id
field, the Scala driver adds the _id
field with an ObjectId value to the new document. See Insert Behavior.
- Mongo Shell
- Compass
- Python
- Java (Sync)
- Node.js
- Other
- PHP
- Motor
- Java (Async)
- C#
- Perl
- Ruby
- Scala
db.inventory.insertOne(
{ item: "canvas", qty: 100, tags: ["cotton"], size: { h: 28, w: 35.5, uom: "cm" } }
)
You can run the operation in the web shell below:
https://mws.mongodb.com/?version=3.6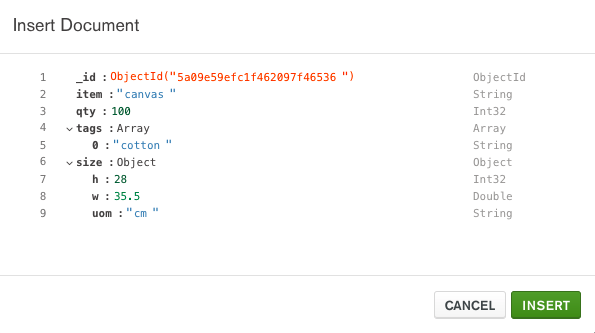
db.inventory.insert_one(
{"item": "canvas",
"qty": 100,
"tags": ["cotton"],
"size": {"h": 28, "w": 35.5, "uom": "cm"}})
Document canvas = new Document("item", "canvas")
.append("qty", 100)
.append("tags", singletonList("cotton"));
Document size = new Document("h", 28)
.append("w", 35.5)
.append("uom", "cm");
canvas.put("size", size);
collection.insertOne(canvas);
await db.collection('inventory').insertOne({
item: 'canvas',
qty: 100,
tags: ['cotton'],
size: { h: 28, w: 35.5, uom: 'cm' }
});
$insertOneResult = $db->inventory->insertOne([
'item' => 'canvas',
'qty' => 100,
'tags' => ['cotton'],
'size' => ['h' => 28, 'w' => 35.5, 'uom' => 'cm'],
]);
await db.inventory.insert_one(
{"item": "canvas",
"qty": 100,
"tags": ["cotton"],
"size": {"h": 28, "w": 35.5, "uom": "cm"}})